Balancing DataTables Responsiveness and Footer Calculations
When using DataTables with the responsive feature enabled, a common challenge arises when attempting to calculate footer sums, as the responsive design converts row data into child rows. In this scenario, the callback function struggles to identify the column location due to the transformation of data into child rows, rendering footer calculations invisible.
To address this issue and enable both responsiveness and footer calculations, a common approach is to use the
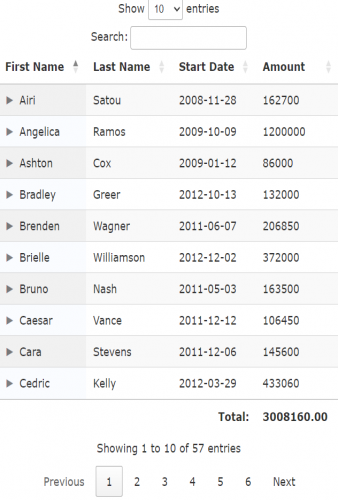
Output While this solution ensures visibility of the footer calculations, it compromises the table's appearance at different resolutions. Finding a balance between responsiveness and accurate footer calculations is crucial for an optimal user experience.
To address this issue and enable both responsiveness and footer calculations, a common approach is to use the
className: 'all'option. However, this solution comes with a drawback, as it forces the column to be visible even at different display resolutions, potentially affecting the table's aesthetics.
<!DOCTYPE html> <html> <head> <script src="https://code.jquery.com/jquery-1.11.3.min.js"></script> <link href="https://cdn.datatables.net/1.13.5/css/jquery.dataTables.css" rel="stylesheet" type="text/css" /> <script src="https://cdn.datatables.net/1.13.5/js/jquery.dataTables.js"></script> <link href="https://cdn.datatables.net/responsive/2.5.0/css/responsive.dataTables.css" rel="stylesheet" type="text/css" /> <script src="https://cdn.datatables.net/responsive/2.5.0/js/dataTables.responsive.js"></script> <meta charset=utf-8 /> <title>DataTables - JS Bin</title> </head> <body> <div class="container"> <table id="quoteTable" class="display nowrap" style="width:100%"> <thead> <tr> <th>First Name</th> <th>Last Name</th> <th>Position</th> <th>Office</th> <th>Start Date</th> <th>Amount</th> </tr> </thead> <tbody> <!-- DataTable content will be loaded here --> </tbody> <tfoot class="tfoot"> <tr> <th></th> <th></th> <th></th> <th></th> <th style="text-align:right">Total:</th> <th></th> </tr> </tfoot> </table> </div> </body> </html> var table = new DataTable('#quoteTable', { fixedHeader: true, responsive: true, processing: true, serverSide: true, ajax: { url: '/ssp/objects.php', type: 'post' }, columns: [ { data: 'first_name' }, { data: 'last_name' }, { data: 'position' }, { data: 'office' }, { data: 'start_date',className: 'all'}, { data: 'salary',className: 'all'}, ], footerCallback: function(row, data, start, end, display) { var api = this.api(); var intVal = function(i) { return typeof i === 'string' ? i.replace(/[$,]/g, '') * 1 : typeof i === 'number' ? i : 0; }; var totalAmount = api.column(5, { search: 'applied' }).data().reduce(function(a, b) { return intVal(a) + intVal(b || 0); }, 0); $(api.column(5).footer()).html(totalAmount.toFixed(2)); }, });and the output will be like:
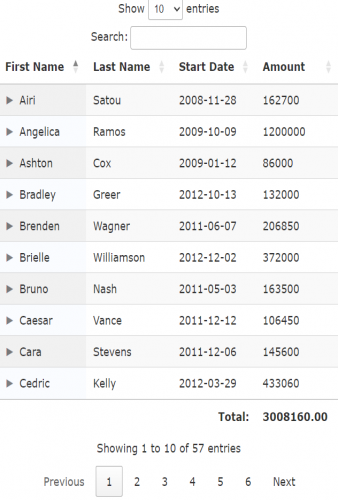
Output While this solution ensures visibility of the footer calculations, it compromises the table's appearance at different resolutions. Finding a balance between responsiveness and accurate footer calculations is crucial for an optimal user experience.
Comments (0)